To concatenate two strings in a PostgreSQL function, you can simply use the concatenate operator (||). For example, you can create a function like this:
CREATE OR REPLACE FUNCTION concat_strings(str1 text, str2 text) RETURNS text AS $$ BEGIN RETURN str1 || str2; END; $$ LANGUAGE plpgsql;
This function takes two text parameters (str1 and str2) and returns the concatenated string of the two input strings. You can then call this function with the two strings you want to concatenate as arguments.
SELECT concat_strings('Hello, ', 'World!');
This will return 'Hello, World!', which is the result of concatenating the two input strings.
Best Oracle Database Books of November 2024
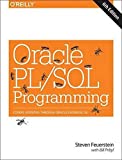
Rating is 4.9 out of 5
Oracle PL/SQL Programming: Covers Versions Through Oracle Database 12c
- O Reilly Media
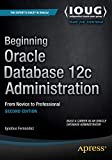
Rating is 4.7 out of 5
Beginning Oracle Database 12c Administration: From Novice to Professional
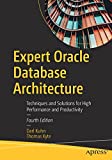
Rating is 4.6 out of 5
Expert Oracle Database Architecture: Techniques and Solutions for High Performance and Productivity
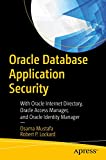
Rating is 4.4 out of 5
Oracle Database Application Security: With Oracle Internet Directory, Oracle Access Manager, and Oracle Identity Manager
What is the correct way to concatenate strings with spaces in PostgreSQL?
In PostgreSQL, you can concatenate strings with spaces using the || operator. Here is the correct way to concatenate strings with spaces in PostgreSQL:
1
|
SELECT 'Hello' || ' ' || 'World';
|
This will output: Hello World
What is the output of using the CONCAT_WS function for string concatenation in PostgreSQL?
The output of using the CONCAT_WS function in PostgreSQL is a string where the specified separator is added between each argument that is concatenated.
For example, if you use the CONCAT_WS function like this:
CONCAT_WS('-', 'John', 'Doe', '123 Main Street')
The output will be:
John-Doe-123 Main Street
How to concatenate strings with a generated sequence in a PostgreSQL function?
To concatenate strings with a generated sequence in a PostgreSQL function, you can use the ||
operator to concatenate the strings and the generate_series
function to generate a sequence of numbers.
Here's an example of how you can concatenate strings with a generated sequence in a PostgreSQL function:
1 2 3 4 5 6 7 8 9 10 11 12 13 |
CREATE OR REPLACE FUNCTION generate_strings_with_sequence(start_num integer, end_num integer) RETURNS text AS $$ DECLARE result text; BEGIN SELECT string_agg('String ' || generate_series(start_num, end_num)::text, ', ') INTO result; RETURN result; END; $$ LANGUAGE plpgsql; SELECT generate_strings_with_sequence(1, 5); |
In this example, the generate_strings_with_sequence
function takes two parameters start_num
and end_num
which specify the start and end of the sequence. The function uses the generate_series
function to generate a sequence of numbers from start_num
to end_num
and then concatenates each number with the string 'String ' using the ||
operator. Finally, the string_agg
function is used to concatenate the resulting strings with a comma separator.
You can then call the generate_strings_with_sequence
function with the desired start and end numbers to concatenate strings with a generated sequence.