In Haskell, you can concatenate variable arguments using the <>
operator from the Data.Monoid
module. This operator is used to combine two monoidal values, which means it is used to concatenate strings in Haskell.
For example, if you have a function that takes a variable number of arguments and you want to concatenate them all into a single string, you can use the <>
operator to do so. Here is an example of how you can concatenate variable arguments in Haskell:
1 2 3 4 5 6 7 8 |
import Data.Monoid concatArgs :: String -> String -> String concatArgs arg1 arg2 = arg1 <> ", " <> arg2 main = do let result = concatArgs "Hello" "World" putStrLn result |
In this example, the concatArgs
function takes two arguments and concatenates them using the <>
operator. You can extend this to concatenate multiple arguments by chaining multiple <>
operations together. For instance, arg1 <> ", " <> arg2 <> ", " <> arg3
.
Top Rated Haskell Books of April 2025
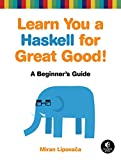
Rating is 4.4 out of 5
Learn You a Haskell for Great Good!: A Beginner's Guide
- No Starch Press
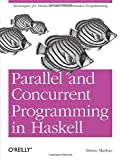
Rating is 4.2 out of 5
Parallel and Concurrent Programming in Haskell: Techniques for Multicore and Multithreaded Programming
- O Reilly Media
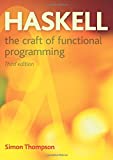
Rating is 4 out of 5
Haskell: The Craft of Functional Programming (International Computer Science Series)
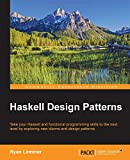
Rating is 3.9 out of 5
Haskell Design Patterns: Take your Haskell and functional programming skills to the next level by exploring new idioms and design patterns
How to concatenate two function outputs in Haskell?
To concatenate two function outputs in Haskell, you can use the ++
operator to concatenate two lists. Here is an example:
1 2 3 4 5 6 7 8 |
function1 :: String -> String function1 str = str ++ "Output from function1" function2 :: String -> String function2 str = str ++ "Output from function2" concatenateOutputs :: String -> String concatenateOutputs input = function1 input ++ function2 input |
In this example, the concatenateOutputs
function takes an input string and concatenates the outputs of function1
and function2
with the input string.
You can also use the ++
operator directly inside another function to concatenate the outputs of two functions, like this:
1 2 |
combinedFunction :: String -> String combinedFunction input = function1 input ++ function2 input |
This will concatenate the outputs of function1
and function2
directly in the combinedFunction
without the need for an intermediate function.
What is the difference between concat and ++ in Haskell?
In Haskell, ++
is used to concatenate two lists, while concat
is used to concatenate a list of lists into a single list.
For example:
1
|
[1, 2, 3] ++ [4, 5, 6] = [1, 2, 3, 4, 5, 6]
|
1
|
concat [[1, 2], [3, 4], [5, 6]] = [1, 2, 3, 4, 5, 6]
|
So, ++
is specifically for concatenating two lists, while concat
is for concatenating a list of lists.
How to concatenate characters in Haskell?
In Haskell, you can concatenate characters by converting them to strings and then using the ++
operator to concatenate the strings. Here is an example that concatenates two characters:
1 2 3 4 5 6 7 8 9 10 11 |
char1 :: Char char1 = 'H' char2 :: Char char2 = 'i' concatenated :: String concatenated = [char1] ++ [char2] main :: IO () main = putStrLn concatenated |
When you run this code, it will output:
1
|
Hi
|
In this example, we convert the characters char1
and char2
to singleton lists [char1]
and [char2]
respectively, then use the ++
operator to concatenate them into a single string concatenated
.