In Haskell, you can convert a list of characters ([[char]]) into a single character by using the head
function, which extracts the first element of a list. Here is an example:
1 2 |
charList = ['a', 'b', 'c', 'd'] singleChar = head charList |
In this example, the variable charList
contains a list of characters, and singleChar
will then be assigned the first character from that list, which is 'a'.
Top Rated Haskell Books of November 2024
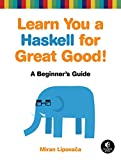
Rating is 4.4 out of 5
Learn You a Haskell for Great Good!: A Beginner's Guide
- No Starch Press
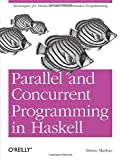
Rating is 4.2 out of 5
Parallel and Concurrent Programming in Haskell: Techniques for Multicore and Multithreaded Programming
- O Reilly Media
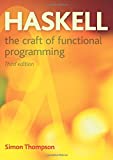
Rating is 4 out of 5
Haskell: The Craft of Functional Programming (International Computer Science Series)
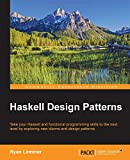
Rating is 3.9 out of 5
Haskell Design Patterns: Take your Haskell and functional programming skills to the next level by exploring new idioms and design patterns
What is the result of converting an empty list of characters to a single char in Haskell?
It is not possible to convert an empty list of characters to a single char in Haskell because an empty list does not have any elements to extract. If you try to do so, it will result in a runtime error.
What is the syntax for converting a list of characters to a single character in Haskell?
The syntax for converting a list of characters to a single character in Haskell is as follows:
1
|
head :: [a] -> a
|
This function takes a list of characters (or any other type) and returns the first element of the list as a single character.
What is the difference between converting a list of characters to a char in Haskell and other functional languages?
In Haskell, a list of characters can be converted to a String
using the concat
function. This function takes a list of strings (each containing a single character) and concatenates them into a single String
. For example:
1 2 |
listToChar :: [Char] -> Char listToChar xs = head (concat [[x] | x <- xs]) |
In other functional languages, such as Lisp or Scheme, the equivalent operation would involve converting the list of characters to a char
type directly. This can be achieved using the car
function, which returns the first element of a list. For example:
1 2 |
(define (listToChar xs) (car xs)) |
Overall, the difference lies in the specific functional programming languages and their syntax and built-in functions for handling lists and characters.
What is the role of type inference when converting a list of characters to a single char in Haskell?
In Haskell, type inference plays a crucial role when converting a list of characters to a single character. Type inference is the process by which the Haskell compiler deduces the data types of expressions in the code based on their usage, without requiring explicit type annotations.
When converting a list of characters to a single character in Haskell, type inference helps ensure that the resulting value has the correct type. For example, if you have a list of characters and you want to convert it to a single character by taking the first element of the list, type inference will automatically infer that the resulting value should be a Char type.
By using type inference, you can write code that is more concise and easier to read, as you don't need to explicitly specify the data types of variables and expressions. Haskell's strong type system and type inference capabilities help prevent type errors and ensure that your code is type-safe.