In Swift, you can declare a variable by using the var keyword followed by the variable name. You can also specify the data type of the variable by using a colon followed by the data type. Optionally, you can provide an initial value for the variable using the equal sign followed by the initial value. For example, you can declare a variable named age of type Int with an initial value of 30 by writing var age: Int = 30. Alternatively, you can omit the data type if you provide an initial value for the variable. The compiler will infer the data type based on the initial value. For example, you can declare a variable named name with an initial value of "John" by writing var name = "John".
Best Swift Books to Read in 2024
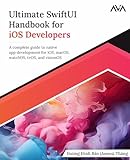
Rating is 4.8 out of 5
Ultimate SwiftUI Handbook for iOS Developers: A complete guide to native app development for iOS, macOS, watchOS, tvOS, and visionOS (English Edition)
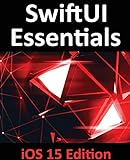
Rating is 4.7 out of 5
SwiftUI Essentials - iOS 15 Edition: Learn to Develop iOS Apps Using SwiftUI, Swift 5.5 and Xcode 13
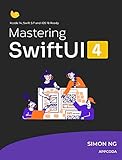
Rating is 4.6 out of 5
Mastering SwiftUI for iOS 16 and Xcode 14: Learn how to build fluid UIs and a real world app with SwiftUI (Mastering iOS Programming and Swift Book 3)
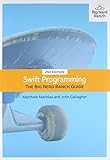
Rating is 4.5 out of 5
Swift Programming: The Big Nerd Ranch Guide (Big Nerd Ranch Guides)
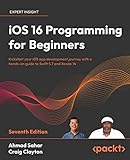
Rating is 4.4 out of 5
iOS 16 Programming for Beginners: Kickstart your iOS app development journey with a hands-on guide to Swift 5.7 and Xcode 14, 7th Edition
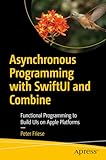
Rating is 4.3 out of 5
Asynchronous Programming with SwiftUI and Combine: Functional Programming to Build UIs on Apple Platforms
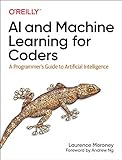
Rating is 4.2 out of 5
AI and Machine Learning for Coders: A Programmer's Guide to Artificial Intelligence
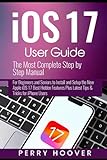
Rating is 4.1 out of 5
iOS 17 User Guide: The Most Complete Step by Step Manual for Beginners and Seniors to Install and Setup the New Apple iOS 17 Best Hidden Features Plus Latest Tips & Tricks for iPhone Users
How to declare a variable as a constant string in Swift?
In Swift, you can declare a constant string variable using the let
keyword followed by the variable name and the string value assigned to it. Here is an example:
1
|
let myConstantString = "This is a constant string"
|
Once you have declared a constant string using let
, you cannot change its value throughout the program. This ensures that the value remains constant and cannot be modified accidentally.
How to assign a value to a variable in Swift?
In Swift, you can assign a value to a variable using the following syntax:
1
|
var variableName: DataType = value
|
For example, to assign a value of 10 to a variable named number
, you would write:
1
|
var number: Int = 10
|
You can also omit the data type if Swift can infer it from the assigned value:
1
|
var anotherNumber = 15
|
In this case, Swift will infer the data type of anotherNumber
as Int
.
How to declare a variable as optional in Swift?
In Swift, you can declare a variable as optional by adding a question mark (?) after the data type when declaring the variable.
For example:
1 2 3 |
var optionalString: String? // Declaring an optional String variable var optionalInt: Int? // Declaring an optional Int variable var optionalDouble: Double? // Declaring an optional Double variable |
By declaring a variable as optional, it means that the variable can either have a value of the specified type or be nil. This allows you to handle situations where a value may be missing or not assigned yet.