To divide ASCII code in PowerShell, you can simply use the division operator (/) or the divide method. This will allow you to divide two ASCII values and perform the necessary calculations. Additionally, you can also use the [math]::DivRem method to get both the quotient and remainder when dividing ASCII codes. This will give you more flexibility in manipulating ASCII values in your PowerShell scripts.
Best PowerShell Books to Read in November 2024
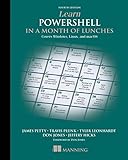
Rating is 5 out of 5
Learn PowerShell in a Month of Lunches, Fourth Edition: Covers Windows, Linux, and macOS
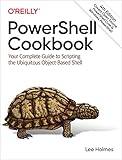
Rating is 4.9 out of 5
PowerShell Cookbook: Your Complete Guide to Scripting the Ubiquitous Object-Based Shell
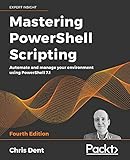
Rating is 4.6 out of 5
Mastering PowerShell Scripting - Fourth Edition: Automate and manage your environment using PowerShell 7.1
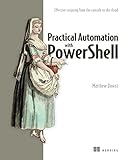
Rating is 4.5 out of 5
Practical Automation with PowerShell: Effective scripting from the console to the cloud
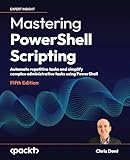
Rating is 4.4 out of 5
Mastering PowerShell Scripting - Fifth Edition: Automate repetitive tasks and simplify complex administrative tasks using PowerShell
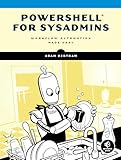
Rating is 4.3 out of 5
PowerShell for Sysadmins: Workflow Automation Made Easy
- Book - powershell for sysadmins: workflow automation made easy
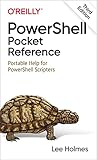
Rating is 4.2 out of 5
PowerShell Pocket Reference: Portable Help for PowerShell Scripters
How to convert ASCII values to characters in PowerShell?
In PowerShell, you can convert ASCII values to characters using the [char]::ConvertFromUtf32()
method. Here's an example of how to convert ASCII values to characters in PowerShell:
1 2 3 4 |
# Convert ASCII value to character $asciiValue = 97 $character = [char]::ConvertFromUtf32($asciiValue) Write-Output $character |
In the above example, the ASCII value 97 corresponds to the character "a". You can replace the $asciiValue
variable with the desired ASCII value you want to convert to a character.
How to find ASCII values of special characters in PowerShell?
To find the ASCII values of special characters in PowerShell, you can use the following command:
1
|
[char]::ConvertToUtf32("special character", 0)
|
Replace "special character" with the character you want to find the ASCII value for. This command will output the ASCII value of the specified special character.
What is the minimum ASCII value in PowerShell?
The minimum ASCII value in PowerShell is 0, which corresponds to the NULL character.
How to calculate the ASCII value for a given string in PowerShell?
You can calculate the ASCII value for a given string in PowerShell by using the [byte]
type accelerator. Here is an example code snippet that demonstrates how to do this:
1 2 3 4 5 6 7 8 9 |
$string = "hello" $asciiValues = @() foreach ($char in $string.ToCharArray()) { $asciiValue = [byte][char]::Parse($char) $asciiValues += $asciiValue } $asciiValues |
This code creates an empty array $asciiValues
to store the ASCII values of individual characters in the string. It then loops through each character in the string, converts it to a byte [byte]
, and adds the ASCII value to the $asciiValues
array.
After running this code snippet, the $asciiValues
array will contain the ASCII values of each character in the string "hello".