To get the datatype of a variable in Julia, you can use the typeof()
function.
For example, if you have a variable x
and you want to know its datatype, you can simply call typeof(x)
.
This will return the datatype of the variable x
, which can be any of the primitive datatypes such as Int64
, Float64
, String
, etc.
You can also use the typeof()
function to get the datatype of an expression or a value by passing it as an argument to the function.
Best Julia Programming Books to Read in November 2024
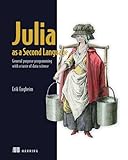
Rating is 5 out of 5
Julia as a Second Language: General purpose programming with a taste of data science
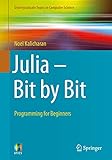
Rating is 4.9 out of 5
Julia - Bit by Bit: Programming for Beginners (Undergraduate Topics in Computer Science)
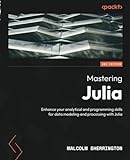
Rating is 4.7 out of 5
Mastering Julia - Second Edition: Enhance your analytical and programming skills for data modeling and processing with Julia
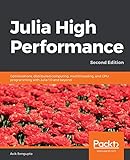
Rating is 4.4 out of 5
Julia High Performance: Optimizations, distributed computing, multithreading, and GPU programming with Julia 1.0 and beyond, 2nd Edition
How to get datatype in Julia for booleans?
In Julia, you can get the datatype for booleans using the typeof()
function.
For example, to get the datatype for a boolean variable b
, you can use the following code:
1 2 |
b = true println(typeof(b)) |
This will output Bool
, which is the datatype for booleans in Julia.
What is the output of getting datatype in Julia?
In Julia, the output of getting the datatype of a variable or value is the type of the variable or value. For example, if you have a variable x
with the value 5
, using the typeof()
function will output Int64
, indicating that x
is an integer of 64 bits.
How to get datatype in Julia for ranges?
In Julia, you can use the eltype()
function to get the data type of elements in a range. Here is an example:
1 2 3 |
r = 1:10 data_type = eltype(r) println(data_type) |
This will output the data type of the elements in the range 1:10
, which is Int64
in this case.
You can also specify the data type when creating a range by using the ::
syntax. For example:
1 2 3 |
r = 1:10::Float64 data_type = eltype(r) println(data_type) |
This will output Float64
as the data type of elements in the range 1.0:10.0
.