In Haskell, you can create a recursive function to effectively loop through a specific action multiple times. By designing the function to call itself within its own definition, you can achieve a looping effect without having to explicitly use traditional looping constructs like for or while loops. This can be done by specifying a base case where the function stops calling itself and returns a final result, ensuring that the loop terminates at a certain condition. This recursive approach allows for concise and elegant code that takes advantage of Haskell's functional programming capabilities.
Top Rated Haskell Books of April 2025
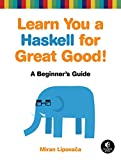
Rating is 4.4 out of 5
Learn You a Haskell for Great Good!: A Beginner's Guide
- No Starch Press
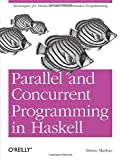
Rating is 4.2 out of 5
Parallel and Concurrent Programming in Haskell: Techniques for Multicore and Multithreaded Programming
- O Reilly Media
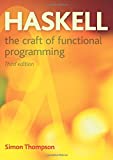
Rating is 4 out of 5
Haskell: The Craft of Functional Programming (International Computer Science Series)
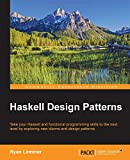
Rating is 3.9 out of 5
Haskell Design Patterns: Take your Haskell and functional programming skills to the next level by exploring new idioms and design patterns
How to pass functions as parameters in Haskell?
To pass a function as a parameter in Haskell, you can simply define the parameter as a function type. Here is an example to demonstrate this:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 |
-- Define a function that takes another function as a parameter applyFunc :: (Int -> Int) -> Int -> Int applyFunc f x = f x -- Define the function that will be passed as a parameter double :: Int -> Int double x = x * 2 -- Call applyFunc with the function double as a parameter result :: Int result = applyFunc double 5 -- Output the result main :: IO () main = print result |
In this example, the applyFunc
function takes a function of type (Int -> Int)
as its first parameter, and an Int
as its second parameter. The double
function is defined as a function that doubles its input. The applyFunc
function is then called with the double
function and the input 5
, resulting in the output 10
.
This is how you can pass functions as parameters in Haskell.
How to define a function in Haskell?
In Haskell, functions are defined using the following syntax:
1 2 |
functionName :: Type1 -> Type2 -> ... -> ReturnType functionName arg1 arg2 ... = <function body> |
Here, functionName
is the name of the function, Type1
, Type2
, etc. are the types of the input arguments, and ReturnType
is the type of the return value. The ->
symbol is used to indicate the function type.
For example, let's define a simple function in Haskell that adds two numbers:
1 2 |
add :: Int -> Int -> Int add x y = x + y |
In this example, add
is the function name, Int -> Int -> Int
specifies that the function takes two Int
arguments and returns an Int
, and x + y
is the body of the function that adds the two arguments.
How to write lambda functions in Haskell?
A lambda function in Haskell is written using the backslash symbol "" followed by the input parameters and then an arrow "->" before the body of the function. Here is the general syntax:
\input -> body
For example, a lambda function that adds two numbers together would be written as:
1
|
(\x y -> x + y)
|
Lambda functions are commonly used in Haskell to create anonymous functions that can be passed as arguments to higher-order functions. Here is a more complex example that uses a lambda function with the map
function to double each element in a list:
1
|
map (\x -> x * 2) [1, 2, 3, 4, 5]
|
This will output [2, 4, 6, 8, 10]
, as each element in the list is doubled using the lambda function (\x -> x * 2)
.