To remove even indexes in Haskell, you can use a combination of the zip
function with a list comprehension. The zip
function can be used to pair each element in the list with its index, and then you can filter out the elements with even indexes using a list comprehension. Here is an example code snippet that demonstrates how to achieve this:
1 2 |
removeEvenIndexes :: [a] -> [a] removeEvenIndexes xs = [x | (x, i) <- zip xs [0..], odd i] |
In this code snippet, the zip
function is used to pair each element in the list xs
with its index, which is generated by the list [0..]
. The list comprehension then filters out the elements with odd indexes by checking if i
is odd.
You can further customize this code snippet based on your specific requirements, such as applying a different filtering condition or using a different indexing scheme.
Top Rated Haskell Books of November 2024
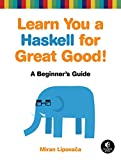
Rating is 4.4 out of 5
Learn You a Haskell for Great Good!: A Beginner's Guide
- No Starch Press
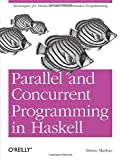
Rating is 4.2 out of 5
Parallel and Concurrent Programming in Haskell: Techniques for Multicore and Multithreaded Programming
- O Reilly Media
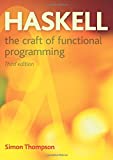
Rating is 4 out of 5
Haskell: The Craft of Functional Programming (International Computer Science Series)
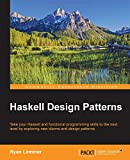
Rating is 3.9 out of 5
Haskell Design Patterns: Take your Haskell and functional programming skills to the next level by exploring new idioms and design patterns
What is a pattern match failure in Haskell?
A pattern match failure in Haskell occurs when a function is defined to match specific patterns (using pattern matching) but the input value does not match any of these patterns. As a result, the function cannot proceed and returns an error, commonly referred to as a pattern match failure. This error typically occurs when a function is defined with incomplete patterns or when the input does not conform to the expected patterns.
How to remove even indexes in JavaScript?
You can remove the even indexes from an array in JavaScript by iterating through the array and checking if the index is even. If it is, you can remove that element from the array. Here is an example of how you can achieve this:
1 2 3 4 5 6 7 8 9 10 |
let arr = [1, 2, 3, 4, 5, 6, 7, 8, 9, 10]; let newArr = []; for (let i = 0; i < arr.length; i++) { if (i % 2 !== 0) { // if index is not even newArr.push(arr[i]); } } console.log(newArr); |
This code will output [2, 4, 6, 8, 10]
, which is the original array with the even indexes removed.
What is the difference between a function and a method in Haskell?
In Haskell, the terms "function" and "method" are often used interchangeably to refer to a piece of code that performs a specific task. However, in a more technical sense:
- Function: In Haskell, a function is a self-contained piece of code that takes input arguments and returns a result. Functions in Haskell are pure, meaning that they always return the same output for the same input and have no side effects. Functions in Haskell are defined using the functionName arguments = result syntax.
- Method: The term "method" is more commonly used in object-oriented programming languages like Java or Python, where a method is a function that is associated with a specific class or object. In Haskell, since it is a functional programming language, the concept of methods is not as prevalent. The closest equivalent in Haskell to a method would be a function that operates on a specific type or data structure.