To reverse a nested list in Haskell, you can use the map
function along with the reverse
function to reverse each individual sublist and then reverse the entire list. This can be accomplished by mapping the reverse
function over the nested list and then applying the reverse
function to the resulting list. This will reverse the nested list in Haskell.
Top Rated Haskell Books of April 2025
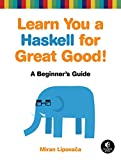
Rating is 4.4 out of 5
Learn You a Haskell for Great Good!: A Beginner's Guide
- No Starch Press
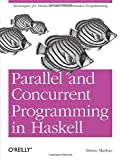
Rating is 4.2 out of 5
Parallel and Concurrent Programming in Haskell: Techniques for Multicore and Multithreaded Programming
- O Reilly Media
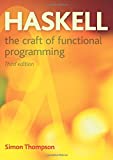
Rating is 4 out of 5
Haskell: The Craft of Functional Programming (International Computer Science Series)
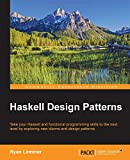
Rating is 3.9 out of 5
Haskell Design Patterns: Take your Haskell and functional programming skills to the next level by exploring new idioms and design patterns
What is the difference between reversing a list and reversing a nested list in Haskell?
Reversing a list in Haskell involves flipping the order of elements within a single list. For example, given the list [1,2,3,4], reversing it would result in [4,3,2,1].
Reversing a nested list in Haskell involves flipping the order of elements within each sub-list, as well as flipping the order of the sub-lists themselves. For example, given the nested list [[1,2], [3,4]], reversing it would result in [[4,3], [2,1]]. In this case, the order of the sub-lists is reversed as well as the order of elements within the sub-lists.
How to reverse a list of lists in Haskell?
You can reverse a list of lists in Haskell by using the reverse
function along with the map
function. Here is an example:
1 2 |
reverseListOfLists :: [[a]] -> [[a]] reverseListOfLists = reverse . map reverse |
In this code, the reverse
function is applied to each individual list in the list of lists using the map
function. Then, the overall list of lists is reversed using the reverse
function.
You can use this function to reverse a list of lists like this:
1 2 3 |
main = do let myList = [[1,2,3], [4,5,6], [7,8,9]] print $ reverseListOfLists myList -- Output: [[9,8,7],[6,5,4],[3,2,1]] |
What is a nested list in Haskell?
A nested list in Haskell is a list that contains other lists as its elements. This means that the elements of the outer list are themselves lists. Nested lists can have multiple levels of nesting, with inner lists containing other lists as elements. Nested lists are commonly used to represent multi-dimensional data structures, such as matrices or trees, in Haskell.