To sum the first elements from lists in Haskell, you can use list comprehension to extract the first elements from each list and then use the sum
function to calculate the sum of these elements. Here's an example code snippet to illustrate this:
1 2 |
sumFirstElements :: Num a => [[a]] -> a sumFirstElements lists = sum [head sublist | sublist <- lists, not (null sublist)] |
In this code, sumFirstElements
is a function that takes a list of lists of numerical values as input. The list comprehension [head sublist | sublist <- lists, not (null sublist)]
iterates through each sublist in the input list, extracts the first element using head
, and filters out any empty sublists using not (null sublist)
. Finally, the sum
function is used to calculate the sum of these first elements.
Top Rated Haskell Books of April 2025
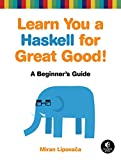
Rating is 4.4 out of 5
Learn You a Haskell for Great Good!: A Beginner's Guide
- No Starch Press
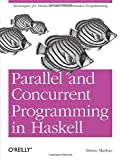
Rating is 4.2 out of 5
Parallel and Concurrent Programming in Haskell: Techniques for Multicore and Multithreaded Programming
- O Reilly Media
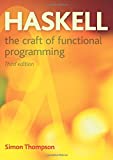
Rating is 4 out of 5
Haskell: The Craft of Functional Programming (International Computer Science Series)
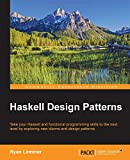
Rating is 3.9 out of 5
Haskell Design Patterns: Take your Haskell and functional programming skills to the next level by exploring new idioms and design patterns
How to sum elements using lambda functions in Haskell?
To sum elements using lambda functions in Haskell, you can use the foldr
function along with a lambda function that adds the elements together. Here's an example of how you can do this:
1 2 3 4 5 6 7 8 9 |
-- Define a list of numbers numbers = [1, 2, 3, 4, 5] -- Use the `foldr` function with a lambda function to sum the elements sumElements = foldr (\x acc -> x + acc) 0 numbers -- Print the result main = do print sumElements |
In this code snippet, we first define a list of numbers. We then use the foldr
function to fold (or reduce) the list using a lambda function that takes two arguments x
and acc
(the current element and the accumulated sum so far) and returns the sum of x
and acc
. We start the fold with an initial accumulator value of 0
.
Finally, we print the result of summing all the elements in the list. When you run this code, you should see the sum of the numbers [1, 2, 3, 4, 5]
printed to the console.
What is a possible alternative approach to summing elements in Haskell?
One possible alternative approach to summing elements in Haskell is to use a higher-order function such as foldr
or foldl
. These functions can be used to apply a binary operation to each element of a list in turn, accumulating a result along the way. For example, you could sum the elements of a list using foldr
like this:
1 2 |
sumElements :: Num a => [a] -> a sumElements xs = foldr (+) 0 xs |
This approach is more concise and idiomatic in Haskell compared to using recursion explicitly. It also allows for more flexibility, as you can easily modify the binary operation and initial accumulator value to compute other types of aggregations.
What is the purpose of summing the first elements from lists in Haskell?
The purpose of summing the first elements from lists in Haskell is to combine or consolidate the values of the first elements of multiple lists into a single value. This can be useful in various scenarios, such as calculating the total of specific elements in a list of numbers or aggregating information from multiple lists. By summing the first elements of lists, you can quickly obtain the desired result without needing to iterate through each list individually.