In Haskell, the map function is used to apply a given function to every element in a list, producing a new list with the results. The general syntax for using map is "map function list". The function provided can be a lambda function, defined function, or built-in function. Map is a higher-order function, meaning it takes a function as an argument. Make sure the function you provide to map takes only one argument, as map will supply the element from the list as the argument. Map is a powerful tool in functional programming for transforming lists without the need for imperative loops.
Top Rated Haskell Books of November 2024
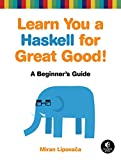
Rating is 4.4 out of 5
Learn You a Haskell for Great Good!: A Beginner's Guide
- No Starch Press
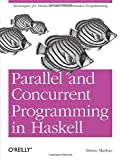
Rating is 4.2 out of 5
Parallel and Concurrent Programming in Haskell: Techniques for Multicore and Multithreaded Programming
- O Reilly Media
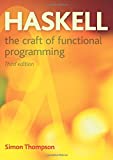
Rating is 4 out of 5
Haskell: The Craft of Functional Programming (International Computer Science Series)
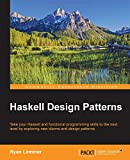
Rating is 3.9 out of 5
Haskell Design Patterns: Take your Haskell and functional programming skills to the next level by exploring new idioms and design patterns
What is the type signature of the lookupAndUpdate function in the map module?
The type signature of the lookupAndUpdate function in the map module would typically be:
lookupAndUpdate :: (a -> Maybe a) -> (a -> a) -> k -> Map k a -> Map k a
This type signature indicates that the function takes three arguments: a function that takes a value of type 'a' and returns a Maybe 'a', a function that takes a value of type 'a' and returns a new value of type 'a', a key of type 'k', and a Map from keys of type 'k' to values of type 'a'. The function returns a new Map with the value corresponding to the given key possibly updated based on the result from the lookup function.
What is the type signature of the map function in the map module?
map :: (a -> b) -> [a] -> [b]
How to perform a lookup followed by an update on a map in Haskell?
To perform a lookup followed by an update on a map in Haskell, you can use the Data.Map
module from the containers
package. Here is an example of how you can achieve this:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 |
import qualified Data.Map as Map -- Define a map myMap :: Map.Map Int String myMap = Map.fromList [(1, "One"), (2, "Two"), (3, "Three")] -- Perform a lookup lookupKey :: Int -> Map.Map Int String -> Maybe String lookupKey key myMap = Map.lookup key myMap -- Update a value updateKey :: Int -> String -> Map.Map Int String -> Map.Map Int String updateKey key value myMap = Map.insert key value myMap -- Lookup a key and update its value lookupAndUpdateKey :: Int -> String -> Map.Map Int String -> Map.Map Int String lookupAndUpdateKey key newValue myMap = case lookupKey key myMap of Just _ -> updateKey key newValue myMap Nothing -> myMap -- Example usage main :: IO () main = do let updatedMap = lookupAndUpdateKey 2 "New Value" myMap print updatedMap |
In this example, we first define a map called myMap
with some key-value pairs. Then, we define a function lookupKey
to perform a lookup on the map for a given key. We also define a function updateKey
to update a value in the map for a given key. Finally, we define a function lookupAndUpdateKey
that first looks up a key in the map and updates its value if it exists, otherwise returns the original map.
You can then use the lookupAndUpdateKey
function to lookup a key in the map and update its value accordingly.
What is the type signature of the fromAscList function in the map module?
fromAscList :: Ord k => [(k, a)] -> Map k a