To create an empty array of arrays in PowerShell, you can use the following syntax:
1
|
$arrayOfArrays = @()
|
This will create an empty array that can hold other arrays. You can then add arrays to this main array as needed by using the +=
operator.
Best PowerShell Books to Read in February 2025
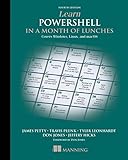
Rating is 5 out of 5
Learn PowerShell in a Month of Lunches, Fourth Edition: Covers Windows, Linux, and macOS
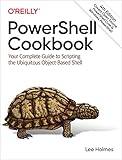
Rating is 4.9 out of 5
PowerShell Cookbook: Your Complete Guide to Scripting the Ubiquitous Object-Based Shell
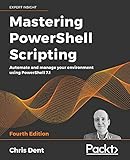
Rating is 4.6 out of 5
Mastering PowerShell Scripting - Fourth Edition: Automate and manage your environment using PowerShell 7.1
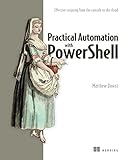
Rating is 4.5 out of 5
Practical Automation with PowerShell: Effective scripting from the console to the cloud
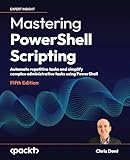
Rating is 4.4 out of 5
Mastering PowerShell Scripting - Fifth Edition: Automate repetitive tasks and simplify complex administrative tasks using PowerShell
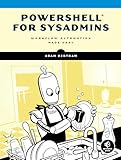
Rating is 4.3 out of 5
PowerShell for Sysadmins: Workflow Automation Made Easy
- Book - powershell for sysadmins: workflow automation made easy
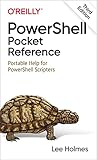
Rating is 4.2 out of 5
PowerShell Pocket Reference: Portable Help for PowerShell Scripters
How to filter an array in PowerShell?
To filter an array in PowerShell, you can use the Where-Object
cmdlet.
Here is an example of how to filter an array of numbers to only include values greater than 5:
1 2 |
$numbers = 1, 3, 6, 8, 10 $filteredNumbers = $numbers | Where-Object { $_ -gt 5 } |
In this example, $filteredNumbers
will contain the values 6, 8, and 10 from the original array $numbers
.
You can adjust the filter criteria inside the script block {}
to suit your specific requirements.
How to reverse an array in PowerShell?
Here's a simple example of how to reverse an array in PowerShell:
1 2 3 4 5 6 7 8 |
# Define an array $array = 1, 2, 3, 4, 5 # Use the Reverse() method to reverse the array [array]::Reverse($array) # Output the reversed array $array |
When you run this code, it will reverse the elements in the array so that the output will be [5, 4, 3, 2, 1]
.
How to merge arrays in PowerShell?
To merge arrays in PowerShell, you can use the "+" operator to combine two or more arrays into one. Here is an example:
1 2 3 4 5 |
$array1 = 1, 2, 3 $array2 = 4, 5, 6 $mergedArray = $array1 + $array2 Write-Output $mergedArray |
This will output:
1 2 3 4 5 6 |
1 2 3 4 5 6 |
Alternatively, you can use the "AddRange()" method to merge arrays. Here is an example:
1 2 3 4 5 6 |
$array1 = 1, 2, 3 $array2 = 4, 5, 6 $array1.AddRange($array2) Write-Output $array1 |
This will also output:
1 2 3 4 5 6 |
1 2 3 4 5 6 |