In Haskell, you can pass the system time to a variable by using the getCurrentTime function from the Data.Time module. This function returns the current system time as a UTCTime value. You can then store this value in a variable using the let keyword or by binding it to a name in a function. Here is an example code snippet that demonstrates how to pass the system time to a variable in Haskell:
1 2 3 4 5 6 |
import Data.Time main = do currentTime <- getCurrentTime let time = show currentTime putStrLn ("Current system time is: " ++ time) |
In this code snippet, we first import the Data.Time module to access the getCurrentTime function. We then use the do notation to execute a sequence of actions. We call getCurrentTime to get the current system time and bind it to the variable currentTime. We then use the let keyword to bind the value of currentTime to the variable time as a string representation. Finally, we print out the current system time using putStrLn.
By following this approach, you can easily pass the system time to a variable in Haskell and use it in your program as needed.
Top Rated Haskell Books of April 2025
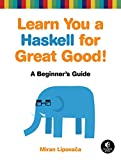
Rating is 4.4 out of 5
Learn You a Haskell for Great Good!: A Beginner's Guide
- No Starch Press
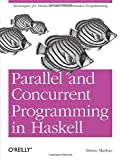
Rating is 4.2 out of 5
Parallel and Concurrent Programming in Haskell: Techniques for Multicore and Multithreaded Programming
- O Reilly Media
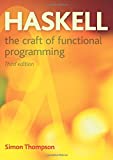
Rating is 4 out of 5
Haskell: The Craft of Functional Programming (International Computer Science Series)
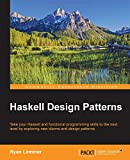
Rating is 3.9 out of 5
Haskell Design Patterns: Take your Haskell and functional programming skills to the next level by exploring new idioms and design patterns
How to access system time and save it in a variable in Haskell?
You can access the system time in Haskell using the getCurrentTime
function from the Data.Time.Clock
module. Here is an example of how to save the system time in a variable:
1 2 3 4 5 6 7 |
import Data.Time.Clock import Data.Time.LocalTime main :: IO () main = do currentTime <- getCurrentTime print currentTime |
In this code snippet, getCurrentTime
function is called to retrieve the current system time and save it in a variable named currentTime
. The print
function is then used to display the current time on the console.
What is the function for fetching system time and assigning it to a variable in Haskell?
In Haskell, you can fetch the system time using the getCurrentTime
function from the Data.Time
module and then covert it to a specific time type if needed. Here is an example of how you can fetch the system time and assign it to a variable:
1 2 3 4 5 |
import Data.Time.Clock main = do currentTime <- getCurrentTime print currentTime |
In this example, getCurrentTime
is used to fetch the current system time as a UTCTime
value. You can then use the currentTime
variable to perform any further operations required with the system time.
What is the function for retrieving system time in Haskell?
The getCurrentTime function from the Data.Time.Clock module is used to retrieve the current system time in Haskell.