To print something to the console in Swift, you can use the print() function. Simply write print() followed by the content you want to display enclosed in quotation marks. For example, if you want to print the message "Hello, World!" to the console, you would write print("Hello, World!"). This will display the message in the Xcode debugger console when you run your Swift code.
Best Swift Books to Read in 2024
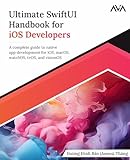
Rating is 4.8 out of 5
Ultimate SwiftUI Handbook for iOS Developers: A complete guide to native app development for iOS, macOS, watchOS, tvOS, and visionOS (English Edition)
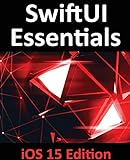
Rating is 4.7 out of 5
SwiftUI Essentials - iOS 15 Edition: Learn to Develop iOS Apps Using SwiftUI, Swift 5.5 and Xcode 13
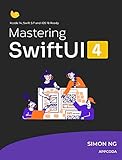
Rating is 4.6 out of 5
Mastering SwiftUI for iOS 16 and Xcode 14: Learn how to build fluid UIs and a real world app with SwiftUI (Mastering iOS Programming and Swift Book 3)
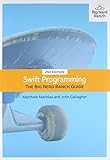
Rating is 4.5 out of 5
Swift Programming: The Big Nerd Ranch Guide (Big Nerd Ranch Guides)
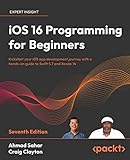
Rating is 4.4 out of 5
iOS 16 Programming for Beginners: Kickstart your iOS app development journey with a hands-on guide to Swift 5.7 and Xcode 14, 7th Edition
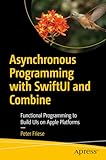
Rating is 4.3 out of 5
Asynchronous Programming with SwiftUI and Combine: Functional Programming to Build UIs on Apple Platforms
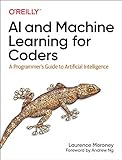
Rating is 4.2 out of 5
AI and Machine Learning for Coders: A Programmer's Guide to Artificial Intelligence
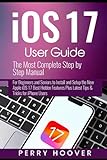
Rating is 4.1 out of 5
iOS 17 User Guide: The Most Complete Step by Step Manual for Beginners and Seniors to Install and Setup the New Apple iOS 17 Best Hidden Features Plus Latest Tips & Tricks for iPhone Users
What is the println() function used for in Swift?
The println()
function in Swift is used for printing a line of text followed by a newline character to the standard output. It is commonly used for debugging purposes to output information or messages to the console during runtime.
How to print a variable along with a string to the console in Swift?
You can print a variable along with a string to the console in Swift by using string interpolation. Here's an example:
1 2 |
let name = "John" print("Hello, \(name)!") |
In this example, the variable name
is inserted into the string using the \(variableName)
syntax within the print
function. This will print "Hello, John!" to the console.
How to print a float to a certain precision in Swift?
In Swift, you can print a float to a certain precision by using the String(format:)
method. Here's an example code snippet to demonstrate how to print a float to two decimal places:
1 2 3 |
let myFloat: Float = 3.14159 let formattedFloat = String(format: "%.2f", myFloat) print(formattedFloat) |
In this code snippet, the String(format:)
method is used with the format specifier %.2f
to specify that the float should be formatted to two decimal places. You can adjust the precision by changing the number after the decimal point in the format specifier.
How to print an array to the console in Swift?
To print an array to the console in Swift, you can use the following code snippet:
1 2 |
let array = [1, 2, 3, 4, 5] print(array) |
This will output the array [1, 2, 3, 4, 5]
to the console.
How to print the value of an optional variable only if it is not nil in Swift?
You can print the value of an optional variable only if it is not nil using optional binding in Swift. Here is an example:
1 2 3 4 5 6 7 |
var optionalValue: Int? = 10 if let value = optionalValue { print("The value is: \(value)") } else { print("The value is nil") } |
In this code snippet, the if let
statement checks if the optional variable optionalValue
has a non-nil value. If it does, the value is unwrapped and assigned to the constant value
, and then it is printed. If the optional variable is nil, then "The value is nil" will be printed instead.