To convert an Int64 to a string in Swift, you can simply use the String constructor that takes an Int64 argument. For example:
let number: Int64 = 123 let numberString = String(number)
This will convert the Int64 number 123 to a string "123". Keep in mind that if you try to convert a very large Int64 number to a string, you may encounter overflow issues. In that case, you can use a number formatter to safely convert the number to a string.
Best Swift Books to Read in 2024
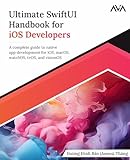
Rating is 4.8 out of 5
Ultimate SwiftUI Handbook for iOS Developers: A complete guide to native app development for iOS, macOS, watchOS, tvOS, and visionOS (English Edition)
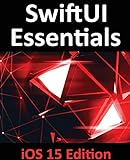
Rating is 4.7 out of 5
SwiftUI Essentials - iOS 15 Edition: Learn to Develop iOS Apps Using SwiftUI, Swift 5.5 and Xcode 13
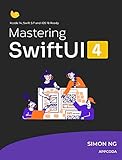
Rating is 4.6 out of 5
Mastering SwiftUI for iOS 16 and Xcode 14: Learn how to build fluid UIs and a real world app with SwiftUI (Mastering iOS Programming and Swift Book 3)
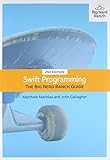
Rating is 4.5 out of 5
Swift Programming: The Big Nerd Ranch Guide (Big Nerd Ranch Guides)
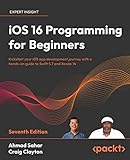
Rating is 4.4 out of 5
iOS 16 Programming for Beginners: Kickstart your iOS app development journey with a hands-on guide to Swift 5.7 and Xcode 14, 7th Edition
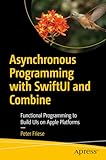
Rating is 4.3 out of 5
Asynchronous Programming with SwiftUI and Combine: Functional Programming to Build UIs on Apple Platforms
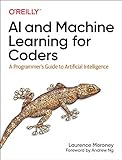
Rating is 4.2 out of 5
AI and Machine Learning for Coders: A Programmer's Guide to Artificial Intelligence
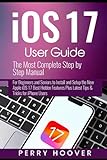
Rating is 4.1 out of 5
iOS 17 User Guide: The Most Complete Step by Step Manual for Beginners and Seniors to Install and Setup the New Apple iOS 17 Best Hidden Features Plus Latest Tips & Tricks for iPhone Users
What is the recommended approach to convert int64 to string in swift?
The recommended approach to convert an Int64 to a string in Swift is to use the String
initializer that takes an integer value as a parameter. Here's an example:
1 2 3 |
let number: Int64 = 123 let stringFromNumber = String(number) print(stringFromNumber) // Output: "123" |
In this example, we use the String
initializer to convert the Int64
number to a string. This is the most straightforward and recommended approach to convert an integer to a string in Swift.
What is the relationship between NSNumberFormatter and converting int64 to string in swift?
NSNumberFormatter is a class in Swift that provides a way to convert numbers to formatted strings and vice versa. When converting an int64 to a string using NSNumberFormatter, you can specify various formatting options such as the number style, currency symbol, decimal separators, and more.
Using NSNumberFormatter to convert an int64 to a string can be useful when you want to format the number in a specific way, such as adding commas as thousand separators or displaying a currency symbol. By using NSNumberFormatter, you can easily achieve this formatting without having to manually manipulate the string representation of the number.
In summary, the relationship between NSNumberFormatter and converting int64 to a string in Swift is that NSNumberFormatter provides a convenient way to format the number as a string with various formatting options.
How to convert int64 to string in swift using the String initializer?
You can convert an Int64
to a String
in Swift by using the String initializer that takes an integer value as an argument. Here is an example code snippet demonstrating how to do this:
1 2 3 |
let intValue: Int64 = 123456789 let stringValue = String(intValue) print(stringValue) // Output: "123456789" |
In this code, we first define an Int64
value intValue
with the value of 123456789
. Then, we simply call the String
initializer with intValue
as an argument to convert it to a String
. Finally, we print out the converted String
value.