To extract the maximum element from a list in Haskell, you can use the maximum
function. This function takes a list of elements and returns the maximum element in that list. You can simply call the maximum
function with your list as an argument to get the maximum element.
Top Rated Haskell Books of April 2025
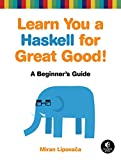
Rating is 4.4 out of 5
Learn You a Haskell for Great Good!: A Beginner's Guide
- No Starch Press
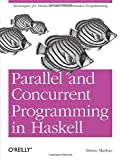
Rating is 4.2 out of 5
Parallel and Concurrent Programming in Haskell: Techniques for Multicore and Multithreaded Programming
- O Reilly Media
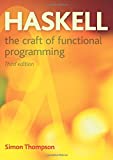
Rating is 4 out of 5
Haskell: The Craft of Functional Programming (International Computer Science Series)
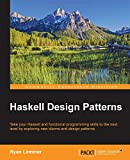
Rating is 3.9 out of 5
Haskell Design Patterns: Take your Haskell and functional programming skills to the next level by exploring new idioms and design patterns
What is the benefit of reversing a list in Haskell?
Reversing a list in Haskell can be beneficial for a few reasons:
- Simplifying code: Reversing a list can make certain algorithms or functions easier to implement and understand. It can often be more straightforward to work with a list in reverse order in certain situations.
- Performance optimization: In some cases, processing a list in reverse order can be more efficient than processing it in the original order. This can be useful for tasks such as searching for an element in a list or applying a function to each element.
- Functional programming principles: Reversing a list can also help programmers practice and understand functional programming principles, such as recursion, pattern matching, and higher-order functions, which are commonly used in Haskell programming.
Overall, reversing a list in Haskell can be a useful tool for solving various programming problems and improving code readability and efficiency.
How to implement a custom function to extract the maximum element from a list in Haskell?
To implement a custom function to extract the maximum element from a list in Haskell, you can use the following approach:
1 2 3 4 5 6 7 8 9 10 11 |
-- Define a custom function to find the maximum element in a list findMax :: (Ord a) => [a] -> a findMax [] = error "List is empty" findMax [x] = x findMax (x:xs) = max x (findMax xs) -- Example usage main = do let myList = [3, 7, 2, 8, 5] let maxElement = findMax myList putStrLn $ "Maximum element in the list is: " ++ show maxElement |
In this implementation, the findMax
function takes a list as input and recursively compares each element in the list to find the maximum element. If the list is empty, an error message is thrown. If the list has only one element, that element is returned as the maximum. Otherwise, the function compares the first element with the maximum of the rest of the list and returns the larger of the two.
You can test this function with a list of integers as shown in the main
function. Simply replace the myList
with your own list and run the program to find the maximum element in the list.
What is the difference between finding the minimum and maximum element in a list in Haskell?
In Haskell, finding the minimum element in a list is done using the minimum
function, which returns the smallest element in the list. On the other hand, finding the maximum element in a list is done using the maximum
function, which returns the largest element in the list.
Here is an example demonstrating how to find the minimum and maximum elements in a list:
1 2 3 4 5 6 7 |
myList = [5, 10, 3, 7, 2] minElement = minimum myList maxElement = maximum myList print minElement -- Output: 2 print maxElement -- Output: 10 |
So, the difference between finding the minimum and maximum element in a list in Haskell is in the functions used (minimum
and maximum
) to retrieve the smallest and largest elements respectively.