To update a list element in Haskell, you can use the update
function from the Data.List
module. This function takes three parameters: the index of the element to be updated, the new value to be inserted, and the original list.
Here is an example of how to update the first element of a list:
1 2 3 4 |
import Data.List myList = [1, 2, 3, 4, 5] updatedList = update 0 10 myList |
In this example, updatedList
will be [10, 2, 3, 4, 5]
, as the first element of myList
has been updated to 10
.
Top Rated Haskell Books of November 2024
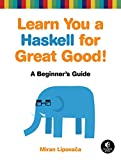
Rating is 4.4 out of 5
Learn You a Haskell for Great Good!: A Beginner's Guide
- No Starch Press
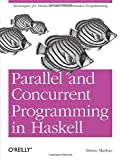
Rating is 4.2 out of 5
Parallel and Concurrent Programming in Haskell: Techniques for Multicore and Multithreaded Programming
- O Reilly Media
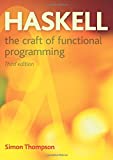
Rating is 4 out of 5
Haskell: The Craft of Functional Programming (International Computer Science Series)
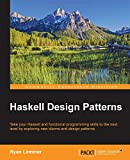
Rating is 3.9 out of 5
Haskell Design Patterns: Take your Haskell and functional programming skills to the next level by exploring new idioms and design patterns
What is the difference between updating a list element in Haskell and replacing a list element?
In Haskell, updating a list element and replacing a list element both involve changing the value of an element in a list. However, there is a subtle difference in how they are achieved.
Updating a list element in Haskell typically involves creating a new list where the desired element has been replaced with a new value. This is done by using functions like take
and drop
to split the original list into two parts, then concatenating the parts with the new value included.
Replacing a list element, on the other hand, involves directly modifying the original list in place. This is not typically done in Haskell, as lists are immutable data structures. Instead, the recommended approach is to create a new list with the desired element replaced, as mentioned above.
In summary, updating a list element in Haskell involves creating a new list with the updated value, while replacing a list element involves directly modifying the original list.
How to update a list element in Haskell using the take function?
In Haskell, you can update a specific element in a list by using the take
function along with list concatenation. Here's an example of how you can update the element at index n
in a list:
1 2 3 4 5 6 7 8 9 10 |
updateElement :: Int -> a -> [a] -> [a] updateElement _ _ [] = [] updateElement n newVal (x:xs) | n == 0 = newVal : xs | otherwise = x : updateElement (n-1) newVal xs main = do let list = [1, 2, 3, 4, 5] let updatedList = updateElement 2 10 list print updatedList |
In this code snippet, the updateElement
function takes three arguments - the index of the element to update, the new value to replace it with, and the original list. It then recursively traverses the list, updating the element at the specified index when it reaches it.
When you run this code, it will output the updated list [1, 2, 10, 4, 5]
, where the element at index 2 (zero-based) has been replaced with the value 10
.
How to update a list element in Haskell using the zip function?
First, let's define a function that updates an element at a specific index in a list using the zip
function:
1 2 3 |
updateElement :: Int -> a -> [a] -> [a] updateElement idx newElement list = zipWith (\i x -> if i == idx then newElement else x) [0..] list |
In this function, idx
is the index of the element we want to update, newElement
is the new value we want to replace it with, and list
is the original list.
Now you can use this function to update an element in a list:
1 2 3 |
let myList = [1, 2, 3, 4, 5] let updatedList = updateElement 2 10 myList -- updatedList is [1, 2, 10, 4, 5] |
In the example above, we're updating the element at index 2 in the list myList
with the value 10. The resulting list updatedList
will have the element at index 2 replaced with 10.