To plot vectors in Python using matplotlib, you can create a new figure and axis using plt.subplots(). Then, you can use the plt.quiver() function to plot the vectors on the axis. This function takes in the starting points, directions, and lengths of the vectors as input parameters. You can customize the appearance of the vectors by specifying parameters such as color, width, and length. Finally, you can use plt.show() to display the plot with the vectors.
Best Matplotlib Books to Read in 2024
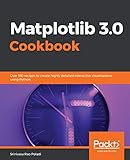
Rating is 4.9 out of 5
Matplotlib 3.0 Cookbook: Over 150 recipes to create highly detailed interactive visualizations using Python
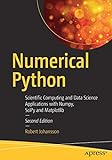
Rating is 4.7 out of 5
Numerical Python: Scientific Computing and Data Science Applications with Numpy, SciPy and Matplotlib
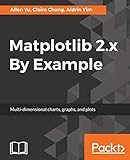
Rating is 4.6 out of 5
Matplotlib 2.x By Example: Multi-dimensional charts, graphs, and plots in Python
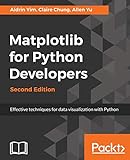
Rating is 4.5 out of 5
Matplotlib for Python Developers: Effective techniques for data visualization with Python, 2nd Edition
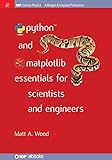
Rating is 4.3 out of 5
Python and Matplotlib Essentials for Scientists and Engineers (Iop Concise Physics)
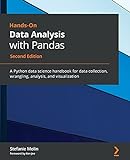
Rating is 4.2 out of 5
Hands-On Data Analysis with Pandas: A Python data science handbook for data collection, wrangling, analysis, and visualization, 2nd Edition
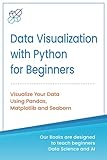
Rating is 4.1 out of 5
Data Visualization with Python for Beginners: Visualize Your Data using Pandas, Matplotlib and Seaborn (Machine Learning & Data Science for Beginners)
How to import matplotlib in a Python script?
To import matplotlib in a Python script, you simply need to add the following line at the beginning of your script:
1
|
import matplotlib.pyplot as plt
|
This will import the matplotlib library and allow you to use its functions and classes in your script. You can then create plots, charts, and graphs using matplotlib's capabilities.
What is matplotlib in Python?
Matplotlib is a plotting library for the Python programming language and its numerical mathematics extension NumPy. It provides a MATLAB-like interface for creating 2D plots and graphs. Matplotlib can be used to create a wide variety of plots, including line plots, scatter plots, bar charts, histograms, and more. It is widely used for data visualization and is a powerful tool for generating high-quality graphics for scientific and engineering applications.
How to display vector components in matplotlib plots?
To display vector components in matplotlib plots, you can use the quiver
function. Here is an example of how to display vector components in a matplotlib plot:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 |
import matplotlib.pyplot as plt import numpy as np # Create a grid of points x = np.linspace(-5, 5, 10) y = np.linspace(-5, 5, 10) X, Y = np.meshgrid(x, y) # Define vector components U = 2 * X V = 2 * Y # Plot vector field plt.quiver(X, Y, U, V, scale=20) plt.xlim(-5, 5) plt.ylim(-5, 5) plt.xlabel('x') plt.ylabel('y') plt.title('Vector Field with Components U = 2x, V = 2y') plt.show() |
In this example, we first create a grid of points using np.linspace
and np.meshgrid
. We then define the vector components U
and V
based on the grid points. Finally, we use the quiver
function to plot the vector field with the specified components. The scale
parameter controls the size of the vectors in the plot.